What are the prerequisites?
1. install the XAMPP server
2. Web browser and the code editor
3. SQL and PHP basics
All the resources and the tutorial video link are provided at the end of the lesson.
Step 1:
Open the XAMPP server and start MySQL and Apache server
Step 3:
Step 4:
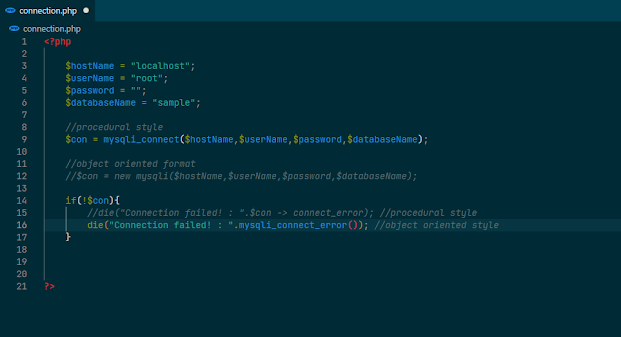
Code explanation :
Note: the lines followed by ‘//’ will not be executed in the PHP script. Those are comments.
You can ignore the Style.
Inside the PHP script, 4 variables are initialized. After that, we can use these variable names anywhere in the PHP script instead of typing the Strings again and again.
After that database is connected using the mysqli_connect() inbuilt function. The function requires 6 parameters and all these parameters are optional. So for this tutorial let’s move with only 4 basic parameters. Also, one of the above 2 formats will be required to connect the database either ‘procedural’ or ‘object oriented’ format.
By the if condition, the error message will be printed for any issue in the database connection. for example Change the database name and see the difference.
Now we are done with the “connection.php” file. Open it in the localhost and check whether is there any error in the database connection.
Step 6:
After that, we are going to do the user interface part using HTML and CSS
Step 7:
function setValue($name){
if(isset($_POST[$name])){
echo $_POST[$name];
}
}
?>
Here we are defining a function called “setValues” and passing the parameter. Inside the function, it will call the value of the POST array using the particular key($name).
After that Inside each input field we are setting the values. After clicking the “Add” button all the values of the input fields will be passed into the POST array.
Let’s move to the next file.
Step 8:
Create a PHP file called “addData.php” and type the below PHP code inside the php tags
By this code, The code will rerun the “connection.php” file here.
If the Add button is clicked then all the details should be in the POST array. By using this condition we are initializing 4 variables again.
Add the MySql syntax to “insert data into a table” and it is inside the insertSql variable.
Here we run the mysqli_query() inbuilt function which requires 2 parameters, one is the database connection and the next one is the MySql query. If it is true then the insert data process is working. So it will redirect to the page “index.php”. Otherwise, it will print the “Connection error” message with the error code.
Inside the “index.php” page we are showing the existing details of the ‘details’ table.
Step 9:
Here is the code for the “index.php” file.
Step 10:
Run the “form.php”, give inputs, and check whether the process is working correctly or not.